This advanced course is designed for developers ready to master Ruby and object-oriented design. Beginning with an in-depth exploration of blocks, procs, and lambdas, you'll learn to write flexible, reusable code.

Early bird sale! Unlock 10,000+ courses from Google, IBM, and more for 50% off. Save today.
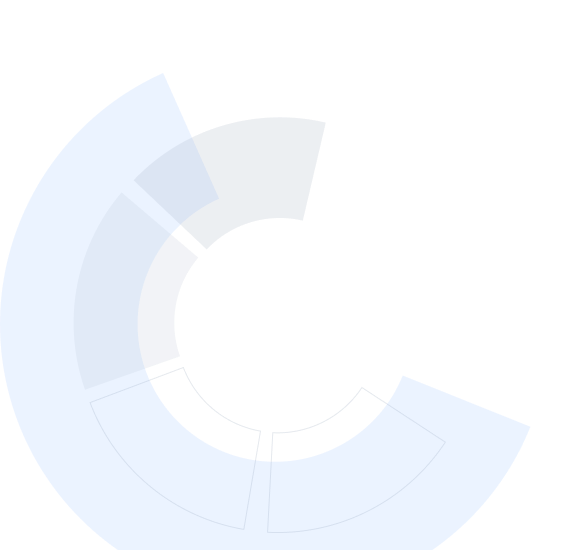
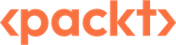
Advanced Ruby Programming and Object-Oriented Design
This course is part of Learn to Code with Ruby Specialization

Instructor: Packt - Course Instructors
Included with
Recommended experience
What you'll learn
Analyze and evaluate the use of blocks, procs, and lambdas to write flexible and reusable code.
Design and create complex class hierarchies and object-oriented structures.
Utilize and implement advanced inheritance techniques and the super keyword in Ruby programming.
Handle and manage file operations, sets, and date/time manipulations effectively.
Skills you'll gain
Details to know

Add to your LinkedIn profile
6 assignments
See how employees at top companies are mastering in-demand skills
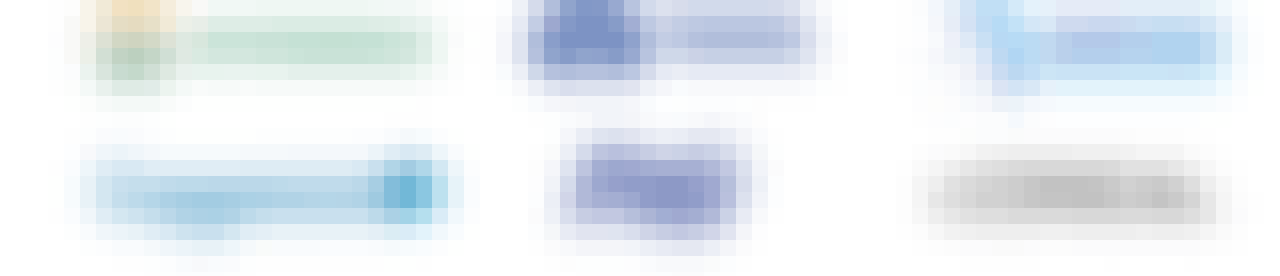
Build your subject-matter expertise
- Learn new concepts from industry experts
- Gain a foundational understanding of a subject or tool
- Develop job-relevant skills with hands-on projects
- Earn a shareable career certificate
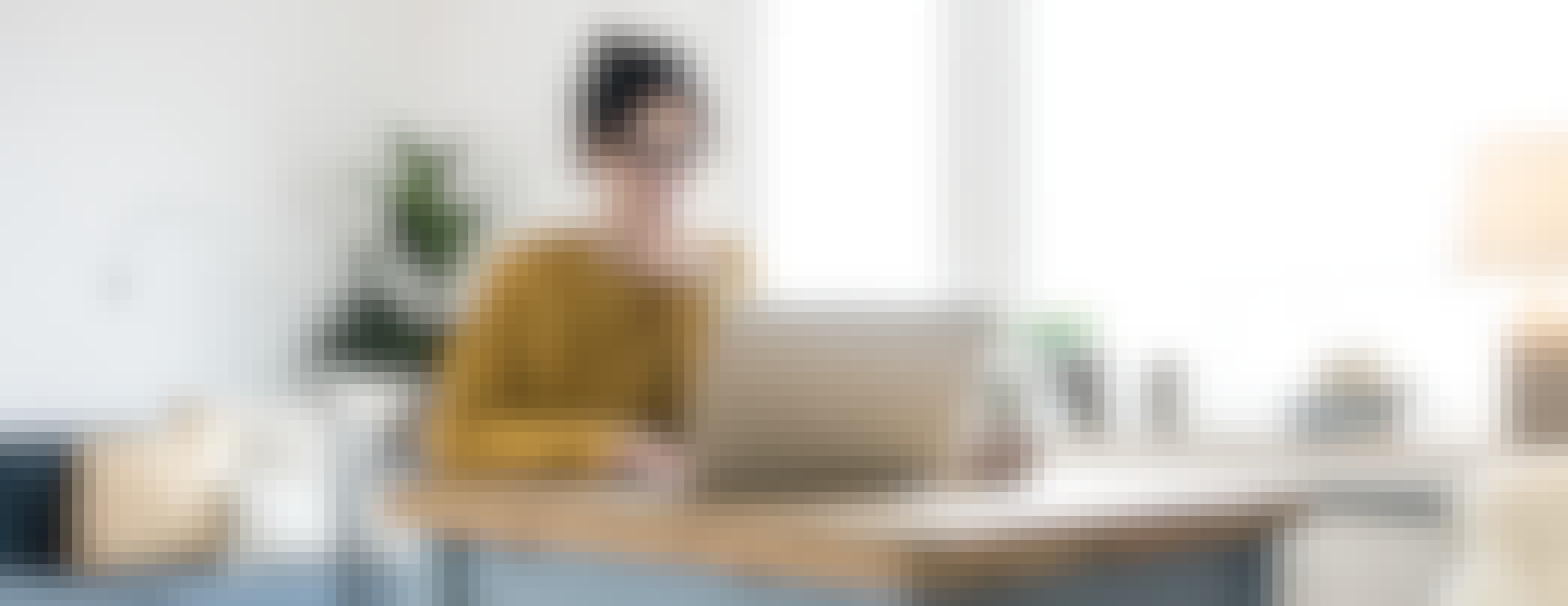
There are 15 modules in this course
In this module, we will delve into the advanced functionalities of blocks, Procs, and lambdas in Ruby. You will learn how to implement and utilize these features to create flexible, reusable, and efficient code. We'll cover key concepts such as the 'yield' keyword, block parameters, and methods with Proc parameters, and provide detailed comparisons between Procs and lambdas. By the end of this section, you'll have a comprehensive understanding of these powerful Ruby tools.
What's included
12 videos2 readings
In this module, we will explore the fundamental principles of object-oriented programming in Ruby by examining classes and objects. You will learn how to define classes, create and use instance variables, and write instance methods to encapsulate functionality. We'll also cover the 'self' keyword, getter and setter methods, and Ruby's shorthand syntax for accessors. By the end of this section, you'll have a solid understanding of how to create and manipulate objects using Ruby classes, setting the foundation for more advanced topics in object-oriented design.
What's included
14 videos
In this module, we will delve deeper into the advanced aspects of Ruby classes. You will learn how to encapsulate class internals with private and protected methods, ensuring controlled access to class functionality. We'll cover how to add validation to setter methods and compute derived values dynamically. Additionally, you'll explore class methods, alternative syntaxes for defining them, and class variables for shared state management. The concept of monkey patching will be introduced, allowing you to extend existing classes with new functionality. This section concludes with a comprehensive review to solidify your understanding of these advanced class concepts.
What's included
10 videos1 assignment
In this module, we will explore the robust feature of keyword arguments in Ruby. You'll learn how to use hashes as initialization arguments to make your methods more flexible and efficient. We'll cover the implementation of required and optional keyword arguments, ensuring your methods can handle varying input needs gracefully. Additionally, we’ll examine the interplay between positional and keyword arguments, providing insights into their effective combination. Through a practical refactoring of the Candidate class, you'll see how keyword arguments can simplify and enhance object initialization. This section concludes with a thorough review to reinforce your understanding of keyword arguments and their benefits.
What's included
6 videos
In this module, we will delve into the powerful concept of inheritance in Ruby. You'll learn to create and manage superclasses and subclasses, understanding the hierarchical relationships that shape object functionality. We'll explore key methods such as 'superclass', 'ancestors', 'is_a?', and 'instance_of?' to trace and verify inheritance hierarchies. You’ll also learn to define exclusive methods in subclasses and override superclass methods. The 'super' keyword will be covered in-depth, along with techniques for defining object equality and leveraging duck typing for dynamic programming. This section concludes with a comprehensive review of all inheritance concepts discussed.
What's included
13 videos
In this module, we will explore various methods for handling input and output operations in Ruby. You'll learn to read from and write to text files, and how to rename and delete files while ensuring their existence. We will also cover how to pass and use command-line arguments via the ARGV array. The module includes a detailed explanation of loading and importing Ruby files using the 'load', 'require', and 'require_relative' methods, highlighting the differences and best practices for each. This section concludes with a comprehensive review to reinforce your understanding of Ruby's file handling and input/output capabilities.
What's included
7 videos1 assignment
In this module, we will explore the powerful concept of modules and mixins in Ruby. You'll learn to define modules with constants and methods, and how to manage multiple modules with identical methods using protected namespaces. We'll cover importing modules into files and utilizing built-in modules like Math, URI, and Net/HTTP. The module will introduce mixins, showcasing how to add methods from modules like Enumerable and Comparable to classes. You'll learn to mix in custom modules, use the 'prepend' and 'extend' keywords, and handle multiple module declarations and nesting within modules. This section concludes with a comprehensive review to solidify your understanding of modules and mixins in Ruby.
What's included
15 videos
In this module, we will delve into the Set data structure in Ruby. You will learn about its properties as a mutable, unordered collection that ensures element uniqueness. We will cover methods to add and delete elements, examining how these methods behave based on the presence or absence of elements within the Set. Additionally, we will explore the Set class's source code on GitHub to gain insights into its implementation. This section concludes with a review to reinforce your understanding of Sets and their practical applications in Ruby programming.
What's included
4 videos
In this module, we will explore the handling of dates and times in Ruby using the Date and Time objects. You will learn to instantiate these objects, perform arithmetic operations to add or subtract time, and compare Time objects using the Comparable module. We'll cover formatting Time objects into strings with the 'strftime' method and parsing strings into Time objects using the 'parse' and 'strptime' methods. This section concludes with a comprehensive review to ensure a solid understanding of managing date and time representations in Ruby for various applications.
What's included
7 videos1 assignment
In this module, we will delve into the powerful tool of regular expressions in Ruby. You'll learn the basics of creating 'Regexp' objects and using simple search methods. We'll explore the 'scan' method to find all matches in a string and practice using symbols like '\d' for digits, '.' for wildcards, and more for custom pattern matching. Anchors and exclusion techniques will be covered to refine your searches. Additionally, we'll learn to use the 'sub' and 'gsub' methods for find-and-replace operations. This section concludes with a review and an introduction to Rubular.com, a helpful resource for experimenting with regular expressions.
What's included
9 videos
In this module, we will explore exception handling in Ruby to manage and respond to errors effectively. You'll learn to use the 'begin' and 'rescue' keywords to define and handle error-prone sections of code. We'll cover capturing error objects with the '=> e' syntax and using multiple rescue clauses for different error types. The 'retry' keyword will be introduced to reattempt code execution, while the 'ensure' keyword will be used for clean-up actions. We'll also discuss how to manually raise exceptions with the 'raise' keyword and define custom error classes for specific error handling. This section concludes with a review to reinforce your understanding of exception handling in Ruby.
What's included
8 videos
In this module, we will immerse ourselves in testing Ruby code using the Minitest framework. Starting with the basics, you'll learn to import the 'minitest/autorun' module, declare test classes, and use common assertions like 'assert_equal'. We'll then test a simple class to put these concepts into practice. You'll learn to set up and tear down test environments using the 'setup' and 'teardown' methods, and validate code behavior with assertions such as 'assert_includes' and 'assert_raises'. Additionally, we will cover how to enhance test messages with custom strings. This section concludes with a comprehensive review of Minitest features and practices to solidify your understanding of testing in Ruby.
What's included
7 videos1 assignment
In this module, we will focus on reading and writing documentation in Ruby. You will learn to navigate the official Ruby documentation, searching for classes and methods while understanding the distinction between class methods ('::') and instance methods ('#'). We'll cover using RDoc to generate webpage documentation for Ruby projects, showing how regular comments can be used to annotate code effectively. By mastering these skills, you'll enhance your ability to document and understand Ruby projects, making your code more maintainable and accessible to others.
What's included
2 videos
In this module, we will explore the world of Ruby Gems, which are pre-written code bundles that can enhance your projects. You'll learn to navigate RubyGems, update the gem program, and install Gems using both 'gem install' and 'bundle install' commands. We'll cover managing project dependencies through a Gemfile to maintain consistency across development environments. Finally, we'll practice using the Faker Gem to generate random data, demonstrating the practical integration of gems into Ruby projects. By the end of this section, you'll be proficient in leveraging gems to add functionality and streamline your Ruby development process.
What's included
4 videos1 assignment
In this final module, we will celebrate the completion of the Advanced Ruby Programming and Object-Oriented Design course. We'll wrap up by summarizing the key learnings and achievements throughout the course. Reflect on the skills acquired, including advanced Ruby techniques, object-oriented design principles, and practical applications. This session provides an opportunity to acknowledge your progress and consider how to apply your new knowledge to future Ruby programming projects.
What's included
1 video1 reading1 assignment
Earn a career certificate
Add this credential to your LinkedIn profile, resume, or CV. Share it on social media and in your performance review.
Instructor

Offered by
Explore more from Software Development
- Status: Free Trial
- Status: Free Trial
- Status: Free Trial
Packt
Coursera Project Network
Why people choose Coursera for their career





Open new doors with Coursera Plus
Unlimited access to 10,000+ world-class courses, hands-on projects, and job-ready certificate programs - all included in your subscription
Advance your career with an online degree
Earn a degree from world-class universities - 100% online
Join over 3,400 global companies that choose Coursera for Business
Upskill your employees to excel in the digital economy
Frequently asked questions
Yes, you can preview the first video and view the syllabus before you enroll. You must purchase the course to access content not included in the preview.
If you decide to enroll in the course before the session start date, you will have access to all of the lecture videos and readings for the course. You’ll be able to submit assignments once the session starts.
Once you enroll and your session begins, you will have access to all videos and other resources, including reading items and the course discussion forum. You’ll be able to view and submit practice assessments, and complete required graded assignments to earn a grade and a Course Certificate.
More questions
Financial aid available,